Cascading Style Sheets (CSS) is a stylesheet language used for describing the look and formatting of a document written in HTML. CSS is used to control the style of a web page, including colors, fonts, layouts, and other design elements.
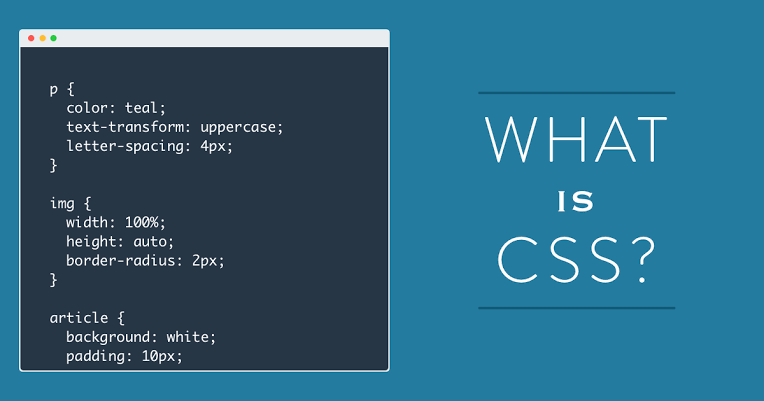
CSS allows you to apply styles to HTML elements, as well as to classes and IDs, which allows you to apply styles to multiple elements at once. You can also use CSS to specify different styles for different media types, such as screen or print.
Using CSS, you can create consistent and professional-looking websites more easily and quickly, because you can separate the content of the HTML document from its presentation. This allows you to make global changes to the design of a website by modifying a single CSS file, rather than making changes to each individual HTML page.
CSS can be written in a separate file with a .css file extension, or it can be included in the HTML file using a style
element in the head
of the document.
How to link css to html
To link a CSS file to an HTML file, you can use a link
element in the head
of the HTML file. The link
element should have a rel
attribute with a value of “stylesheet” and an href
attribute with the URL of the CSS file.
Here’s an example:
<head>
<link rel="stylesheet" href="/path/to/styles.css">
</head>
You can also include multiple CSS files by using multiple link
elements.
If the CSS file is in the same directory as the HTML file, you can use a relative path for the href
attribute. For example:
<head>
<link rel="stylesheet" href="styles.css">
</head>
You can also include CSS styles directly in the HTML file using a style
element in the head
of the HTML file.
<head>
<style>
/* your CSS styles here */
</style>
</head>
Which css attribute would change an element’s font color ?
To change an element’s font color in CSS, you can use the color
attribute. Here’s an example:
.my-element {
color: blue;
}
This will change the font color of all elements with the class “my-element” to blue.
You can also use the color
attribute to change the color of specific HTML elements, like this:
p {
color: green;
}
This will change the font color of all p
(paragraph) elements to green.
You can specify the color using a variety of formats, such as a named color (like “blue” or “green”), a hexadecimal value (like “#0000FF” for blue), or a RGB value (like “rgb(0, 0, 255)” for blue).
.my-element {
color: #0000FF; /* blue */
}
p {
color: rgb(0, 128, 0); /* green */
}
How to center text in css
To center text using CSS, you can use the text-align
property and set it to “center”. Here’s an example:
.my-element {
text-align: center;
}
This will center the text of all elements with the class “my-element”.
You can also use the text-align
property to center specific HTML elements, like this:
p {
text-align: center;
}
This will center the text of all p
(paragraph) elements.
You can also use the margin
property to center block-level elements. For example:
.my-element {
margin: 0 auto;
width: 50%;
}
This will center the element with the class “my-element” within its parent element. The element will also have a width of 50%.
Note that the text-align
property only works on inline elements and elements with a specified width. To center block-level elements, you must use the margin
property as shown above.
How to center an image in css
To center an image using CSS, you can use the margin
property and set it to “auto”. Here’s an example:
img {
display: block;
margin: auto;
}
This will center the image within its parent element. The display: block
property is used to specify that the image should be treated as a block-level element, which allows you to use the margin: auto
property to center it.
You can also use the text-align
property to center an image, like this:
.my-element {
text-align: center;
}
img {
display: inline;
}
This will center the image within the element with the class “my-element”. The display: inline
property is used to specify that the image should be treated as an inline element, which allows it to be centered using the text-align
property.
Note that the text-align
property only works on inline elements and elements with a specified width. To center block-level elements, you must use the margin
property as shown in the first example.
How to add background image in css
To add a background image to an element in CSS, you can use the background-image
property. Here’s an example:
.my-element {
background-image: url('/path/to/image.jpg');
}
This will add the image located at /path/to/image.jpg
as the background image for all elements with the class “my-element”.
You can also use the background-image
property to add a background image to a specific HTML element, like this:
body {
background-image: url('/path/to/image.jpg');
}
This will add the image as the background image for the body
element.
You can use the background-repeat
property to specify whether the background image should be repeated, and the background-position
property to specify the position of the background image.
For example:
.my-element {
background-image: url('/path/to/image.jpg');
background-repeat: no-repeat;
background-position: center;
}
This will add the image as the background image for all elements with the class “my-element”, and it will not repeat. The image will be centered within the element.
How to change font in css
To change the font in CSS, you can use the font-family
property. Here’s an example:
.my-element {
font-family: Arial, sans-serif;
}
This will change the font of all elements with the class “my-element” to Arial. If the user’s device does not have Arial installed, it will use a similar sans-serif font.
You can also use the font-family
property to change the font of specific HTML elements, like this:
p {
font-family: Times New Roman, serif;
}
This will change the font of all p
(paragraph) elements to Times New Roman. If the user’s device does not have Times New Roman installed, it will use a similar serif font.
You can specify multiple fonts as a “font stack”, with the browser using the first available font. This is useful in case the user’s device does not have a specific font installed.
For example:
.my-element {
font-family: Arial, Helvetica, sans-serif;
}
This will use Arial if available, or fall back to Helvetica if Arial is not available, or to a similar sans-serif font if neither Arial nor Helvetica is available.
You can also use the font-size
, font-weight
, font-style
, and other font-related properties to style the text further.
What is em in css
In CSS, the em
unit is a relative unit that is used for specifying font sizes, as well as other lengths in an element’s layout.
An ‘em
‘ is equal to the font size of the element itself. For example, if the font size of an element is 16px
, then ‘1em
‘ is equal to 16px
. If the font size of a child element is set to ‘2em
‘, then the child element’s font size will be 32px
(2 * 16px).
The em
unit is useful because it allows you to specify font sizes that are relative to the font size of the parent element. This makes it easier to create scalable and responsive designs, because you can adjust the font size of the parent element and have all of the child elements scale accordingly.
You can also use the rem
unit, which stands for “root em
“. The rem
unit is similar to the em
unit, but it is based on the font size of the root element (i.e., the html
element) rather than the font size of the element itself.
Here’s an example of using the ‘em
‘ and rem
units in CSS:
html {
font-size: 16px;
}
.my-element {
font-size: 1.5em; /* 1.5 * 16px = 24px */
line-height: 2rem; /* 2 * 16px = 32px */
}
In this example, the font size of the element with the class “my-element” is set to 1.5em
, which is equal to 24px
(1.5 * 16px). The line height is set to 2rem
, which is equal to 32px
(2 * 16px).
How to resize an image in css
To resize an image in CSS, you can use the width
and height
properties. Here’s an example:
img {
width: 100px;
height: 200px;
}
This will resize all img
elements to a width of 100px
and a height of 200px
.
You can also use the max-width
and max-height
properties to set the maximum size of the image. This is useful for preserving the aspect ratio of the image when resizing.
For example:
img {
max-width: 100px;
max-height: 200px;
}
This will resize the image to a maximum width of 100px
and a maximum height of 200px
, while preserving the aspect ratio of the image.
You can also use the width
and height
properties to set the size of a specific HTML element, like this:
#my-element {
width: 300px;
height: 100px;
}
This will resize the element with the ID “my-element” to a width of 300px
and a height of 100px
.
Note that resizing an image in CSS may cause the image to appear distorted or pixelated, depending on the original size and aspect ratio of the image. It is generally a better practice to resize the image using an image editing tool before including it in your HTML.